Sign off your commits
1
2
|
git commit --signoff --message 'This is my commit message'
git commit -s -m "This is my commit message"
|
Submitting a PR from a specific branch
To resync your main
branch with all the changes.
1
2
3
4
|
$ git fetch upstream
$ git checkout main
$ git merge upstream/main
$ git push origin main
|
If there is no upstream
1
2
3
|
$ git remote -v
> origin https://github.com/YOUR_USERNAME/YOUR_FORK.git (fetch)
> origin https://github.com/YOUR_USERNAME/YOUR_FORK.git (push)
|
1
|
$ git remote add upstream https://github.com/ORIGINAL_OWNER/ORIGINAL_REPOSITORY.git
|
1
2
3
4
5
|
$ git remote -v
> origin https://github.com/YOUR_USERNAME/YOUR_FORK.git (fetch)
> origin https://github.com/YOUR_USERNAME/YOUR_FORK.git (push)
> upstream https://github.com/ORIGINAL_OWNER/ORIGINAL_REPOSITORY.git (fetch)
> upstream https://github.com/ORIGINAL_OWNER/ORIGINAL_REPOSITORY.git (push)
|
Now your main
branch has the latest code. Create a new branch called ISSUE1
now and you can fix the bug and submit a PR from that branch.
To list branches
Create a new branch named ISSUE1
Switch over to the branch ISSUE1
when you want to add new commits to it.
Once you are on the ISSUE1
branch, you can start adding commits to it.
1
2
|
$ git add .
$ git commit -m "commit for ISSUE1"
|
To merge commits into the main
branch, let’s now switch over to the main
branch.
Now that ISSUE1
has been successfully merged with main
, we can delete it.
1
|
$ git branch -d <branchname>
|
Personal access token (PAT)
You can also use personal access tokens to authenticate against Git over HTTP. They are the only accepted password when you have Two-Factor Authentication (2FA) enabled.
First, you need to create a personal access token (PAT). This is described here:
1
2
3
|
$ git clone https://github.com/user-or-organisation/myrepo.git
Username: <my-username>
Password: <my-personal-access-token>
|
1
2
3
|
$ git push https://github.com/user-or-organisation/myrepo.git
Username: <my-username>
Password: <my-personal-access-token>
|
Switch git repository to a particular commit
If you want to create a new branch to retain commits you create, you may do so (now or later) by using -b with the checkout command again. Example:
1
2
|
git checkout -b <new-branch-name>
git checkout -b newbranch 6e559cb95
|
To get back to main
branch commit
Rename a Tag on Git
1
2
3
4
|
git tag new old
git tag -d old
git push origin :refs/tags/old
git push --tags
|
Delete a Tag on Git
1
2
|
git push origin :refs/tags/tagname
git tag --delete tagname
|
Delete a submodule
Remove the submodule entry from .git/config
1
|
git submodule deinit -f path/to/submodule
|
Remove the submodule directory from the superproject’s .git/modules
directory
1
|
rm -rf .git/modules/path/to/submodule
|
Remove the entry in .gitmodules and remove the submodule directory located at path/to/submodule
1
|
git rm -f path/to/submodule
|
See all Branches
Create a Branch from previous commit
1
|
git branch branchname <sha1-of-commit>
|
Push the new Branch to server
1
|
git push origin BRANCH_NAME
|
Clone a specific Git branch
1
|
git clone -b <branch> <remote_repo>
|
Clone including submodules
1
|
git clone --recurse-submodules -j8 <remote_repo>
|
Rename the master branch name
1
2
3
4
5
|
git branch -m old_branch new_branch # Rename the branch locally
git branch -m new_branch # Rename the currently checked out branch
git push origin new_branch
# Then go to GitHub UI settings/options, if the old branch was 'master', change 'Default Branch' to another branch that is not 'master'. Then
git push origin :old_branch # Delete the old branch
|
Rename a local and remote branch
Rename your local branch
1
2
3
4
|
#If you are on the branch you want to rename
git branch -m new-name
#If you are on a different branch
git branch -m old-name new-name
|
Delete the old-name remote branch and push the new-name local branch
1
|
git push origin :old-name new-name
|
Reset the upstream branch for the new-name local branch
1
2
|
#Switch to the branch and then
git push origin -u new-name
|
Delete a Git Branch Both Locally and Remotely
1
2
3
4
5
6
7
|
// delete branch locally
git branch -d localBranchName
// delete branch remotely
git push origin --delete remoteBranchName
// or shorter
git push origin :remoteBranchName
|
Prune a branch locally available in “remotes/”
Deletes all stale remote-tracking branches under . These stale branches have already been removed from the remote repository referenced by , but are still locally available in “remotes/”.
With –dry-run option, report what branches will be pruned, but do not actually prune them.
1
2
3
|
#to preview what branches will be removed
git remote prune origin --dry-run
git remote prune origin
|
Moving uncommitted changes to a new branch
1
2
|
git checkout -b newBranch
git status
|
Revert uncommitted changes including files and folders
1
2
3
4
5
6
|
# Revert changes to modified files.
git reset --hard
# Remove all untracked files and directories.
# `-f` is `force`, `-d` is `remove directories`
git clean -fd
|
Pull rebase
Rebase your local changes on the newest codebase.
Remove a Commit That Is Already Pushed to Git
Firstly, find out the comit that you want to revert back to.
For example, commit 7f6d03 was before the 2 wrongful commits.
The + is interpreted as forced push.
Force push that commit as the new main:
1
|
git push origin +7f6d03:main
|
You can also use git reset to undo things. Then force push.
1
2
3
4
5
|
git reset 7f6d03 --hard
git push origin HEAD -f
# or
git push origin main --force
|
If others are working on the same branch, they will need to synchronize their local branches after you force push by running:
1
2
|
git fetch
git reset --hard origin/<branch_name>
|
Remove an unpushed outgoing commit
Reset local repository to be like the remote repo
Setting your branch to exactly match the remote branch can be done in two steps:
1
2
|
git fetch origin
git reset --hard origin/main
|
If you want to save your current branch’s state before doing this (just in case), you can do:
1
2
|
git commit -a -m "Saving my work, just in case"
git branch my-saved-work
|
Retrieve the remote git address
Retrieve the active commit
1
|
git log --pretty=format:'%h' -n 1
|
Changing the latest Git commit message
1
2
|
git commit --amend -m "New message"
git push --force repository-name branch-name
|
Alias
To create shortcut for long commands. Below defines loagd
instead of log --oneline --all --graph --decorate
. Config file location can be --global
, --system
, --local
, --file
, --blob
.
1
|
git config --global alias.loagd "log --oneline --all --graph --decorate"
|
To list the content of git config
1
|
git config --global --list
|
To find the place of a command
1
|
git config --show-origin alias.loagd
|
To see the content of a command
1
|
git config --get alias.loagd
|
Merge Strategies
References
Explicit Merge: Creates a new merge commit. (--no-ff
)
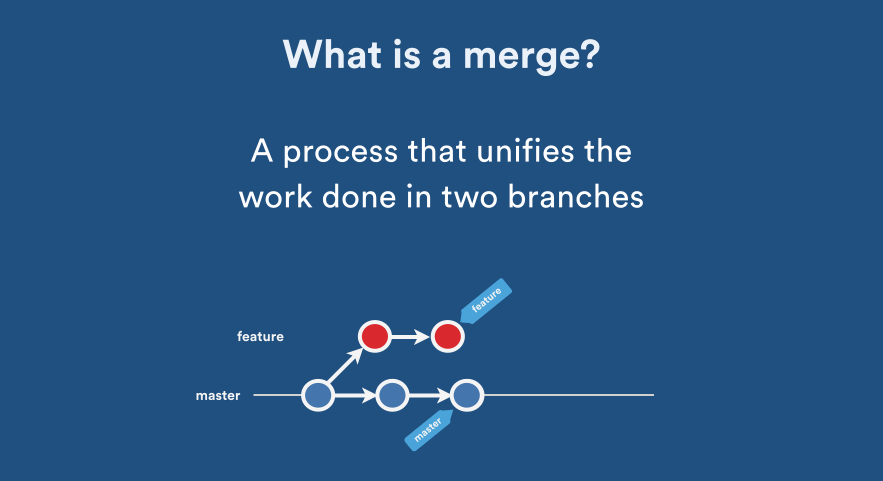
Workflow
1
2
3
4
5
6
7
8
|
git checkout -b development
(do your work on "development" branch)
git status
git commit -am "updates in development branch"
git checkout main
git merge --no-ff development
git branch -d development
git push origin main
|
Fast Forward Merge: Forward rapidly, without creating a new commit: (--ff
)
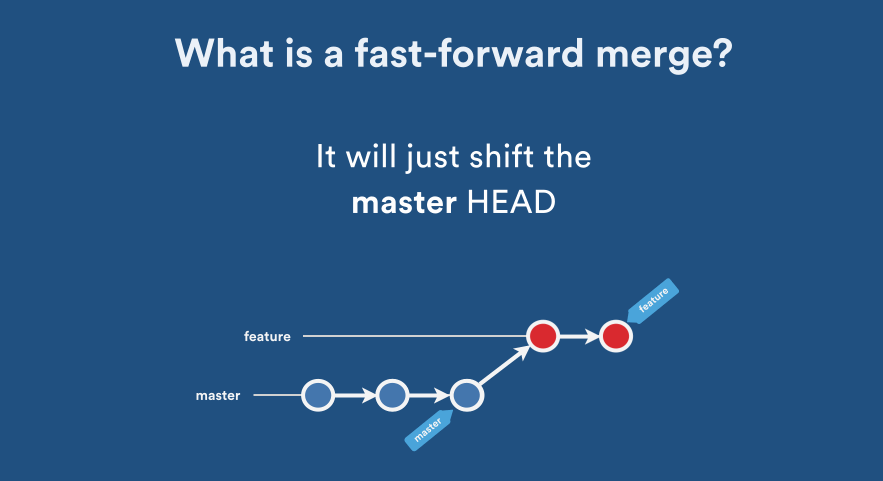
Rebase: Establish a new base level:
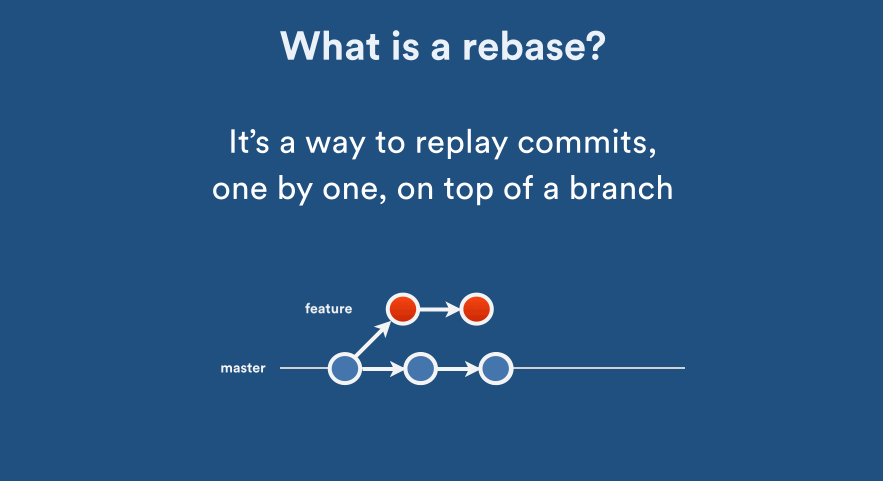
Squash on Merge: Crush or squeeze (something) with force so that it becomes flat: (--squash
)
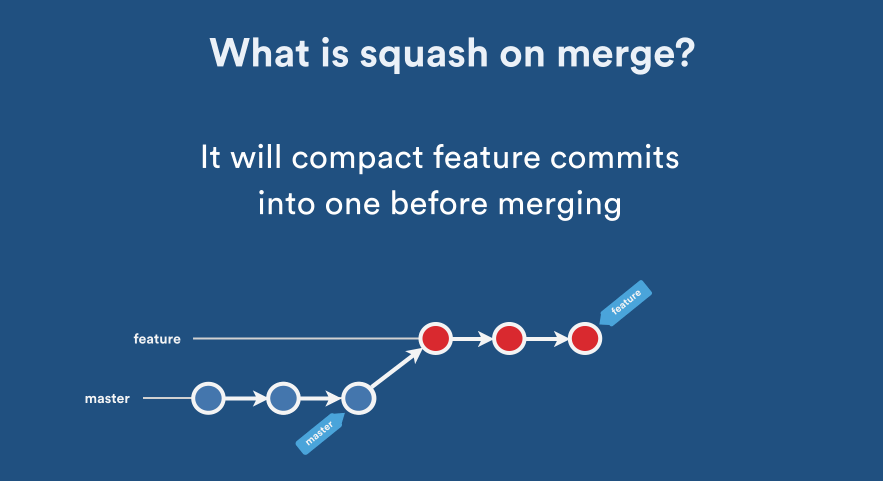
Common commands
- git init: initializes a new
- git add: stages files
- git commit: commits staged files
- git branch: shows current branches
- git branch [branch]: creates new topic branch
- git checkout [branch]: switched HEAD to specified branch
- git merge [branch]: merges [branch] into current branch
- git diff: shows diff of unstages changes
- git diff –cached: shows diff of staged changes
- git log: shows history of current branch
- git log m/[codeline]: shows commits that are not pushed
Useful commands
Listing the N=10 largest files
1
|
git ls-tree -r -t -l --full-name HEAD | sort -n -k 4 | tail -n 10
|